Quick Start
Add GitHub Copilot-style AI completions to your Monaco Editor in in just 3 simple steps 🚀
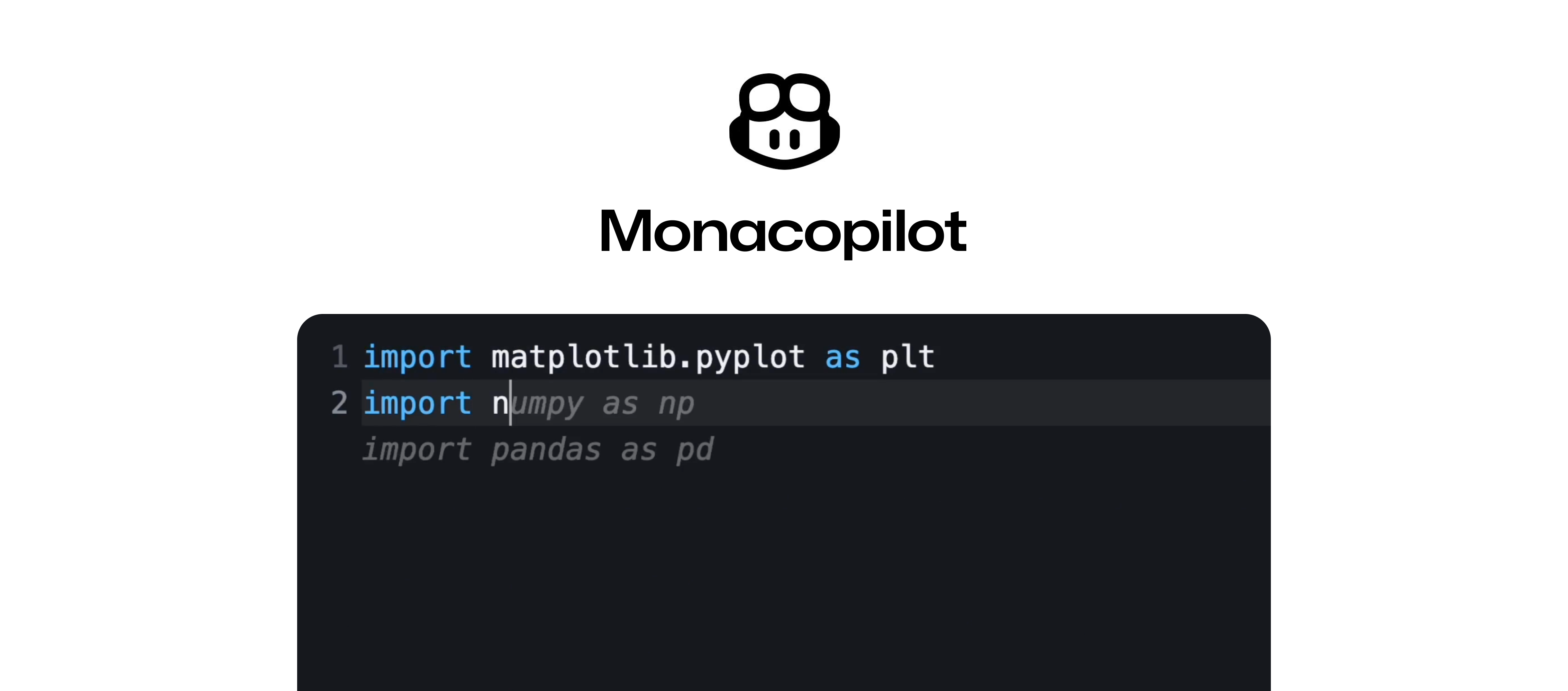
Install the package
npm install monacopilot
yarn add monacopilot
pnpm add monacopilot
bun add monacopilot
<script src="https://unpkg.com/monacopilot@1.1.24/dist/index.global.js"></script>
<!-- or -->
<script src="https://cdn.jsdelivr.net/npm/monacopilot@1.1.24/dist/index.global.js"></script>
Register the AI completion to your editor
In your frontend code:
import * as monaco from 'monaco-editor';
import {registerCompletion} from 'monacopilot';
const editor = monaco.editor.create(document.getElementById('container'), {
language: 'javascript',
});
registerCompletion(monaco, editor, {
language: 'javascript',
// Your API endpoint for handling completion requests
endpoint: 'https://your-api-url.com/code-completion',
});
Create your completion API handler
Create an API handler for the endpoint (e.g. /code-completion
) you provided in the registerCompletion
function to handle completion requests from the editor.
You can use any JavaScript runtime or Node.js framework that can handle HTTP requests and return JSON responses for completions. Below is an example implementation using Express.js and Bun.
import 'dotenv/config';
import cors from 'cors';
import express from 'express';
import {CompletionCopilot} from 'monacopilot';
const app = express();
app.use(cors());
app.use(express.json());
const copilot = new CompletionCopilot(process.env.MISTRAL_API_KEY, {
provider: 'mistral',
model: 'codestral',
});
app.post('/code-completion', async (req, res) => {
const completion = await copilot.complete({body: req.body});
res.json(completion);
});
app.listen(process.env.PORT || 3000);
import {CompletionCopilot} from 'monacopilot';
const copilot = new CompletionCopilot(process.env.MISTRAL_API_KEY, {
provider: 'mistral',
model: 'codestral',
});
Bun.serve({
port: process.env.PORT || 3000,
routes: {
'/completion': {
POST: async req => {
const body = await req.json();
const completion = await copilot.complete({body});
return Response.json(completion);
},
},
},
});
Obtain your Mistral API Key from the Mistral AI Console.
Monacopilot supports multiple AI providers and models. For details on available options and configuration, see the Changing the Provider and Model documentation.
That's it! Your Monaco Editor now has AI-powered completions! 🎉
INFO
The example above uses Express.js. For implementations in other languages like Python, Go, or Ruby, see our Cross-Language API Handler Implementation guide.